如何解密平台证书
更新时间:2024.12.20为了保证安全性,微信支付在回调通知和平台证书下载接口中,对关键信息进行了AES-256-GCM加密,商户收到报文后,要解密出明文,APIv3密钥是解密时使用的对称密钥。本章节详细介绍了加密报文的格式,以及如何进行解密。
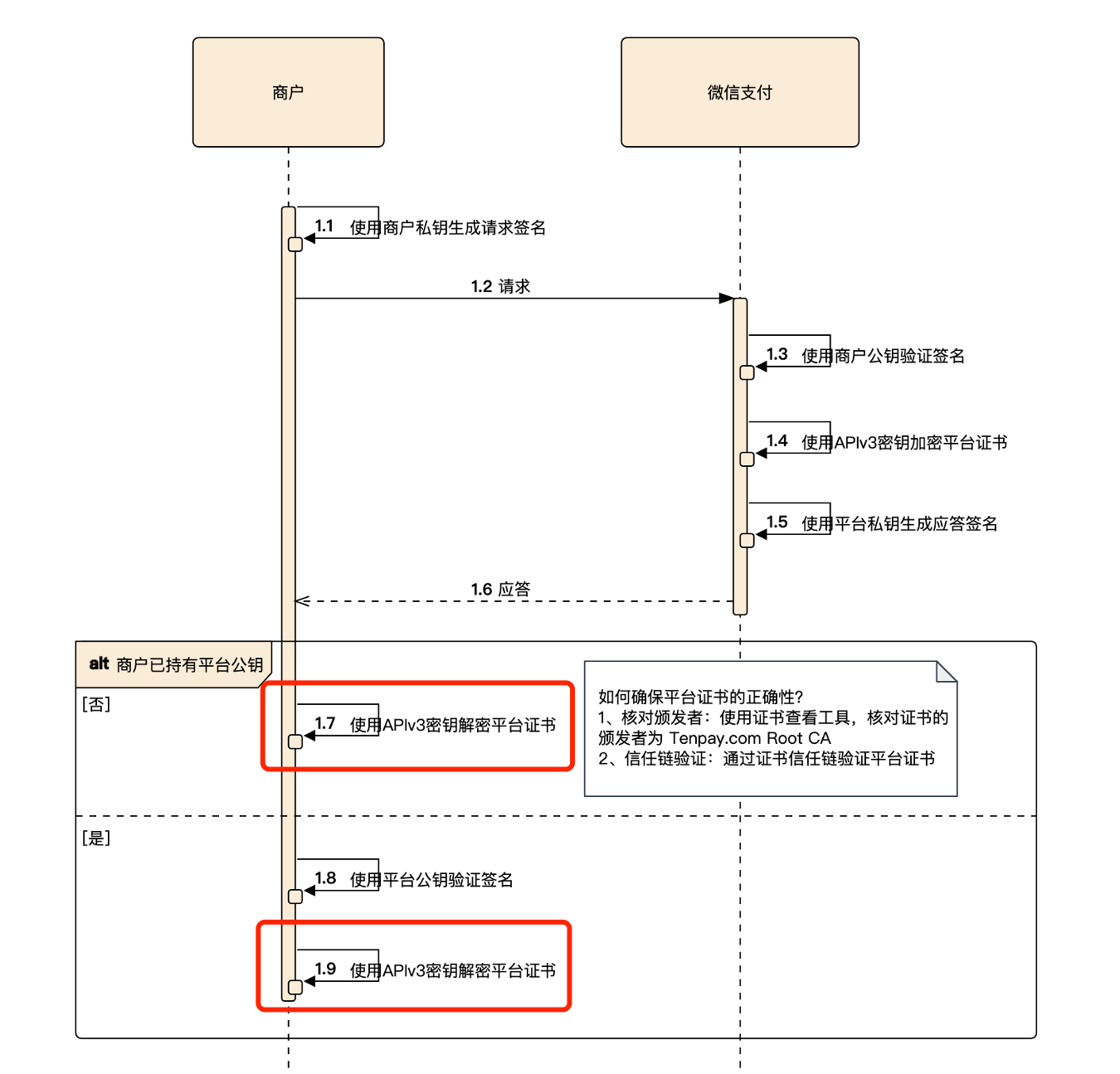
# 1. 加密报文格式
AES-GCM是一种NIST标准的认证加密算法, 是一种能够同时保证数据的保密性、 完整性和真实性的一种加密模式。
对于加密的数据,我们使用了一个独立的JSON对象来表示。为了方便阅读,示例做了Pretty格式化,并加入了注释。
1{2 "original_type": "transaction", // 加密前的对象类型3 "algorithm": "AEAD_AES_256_GCM", // 加密算法45 // Base64编码后的密文6 "ciphertext": "...",7 // 加密使用的随机串初始化向量)8 "nonce": "...",9 // 附加数据包(可能为空)10 "associated_data": ""11}
注意
加密的随机串,跟签名时使用的随机串没有任何关系,是不一样的。
# 2. 解密
算法接口的细节,可以参考rfc5116 (opens new window)。
大部分编程语言(较新版本)都支持了AEAD_AES_256_GCM。开发者可以参考下列的示例,了解如何使用您的编程语言实现解密。
示例代码
文档是否有帮助